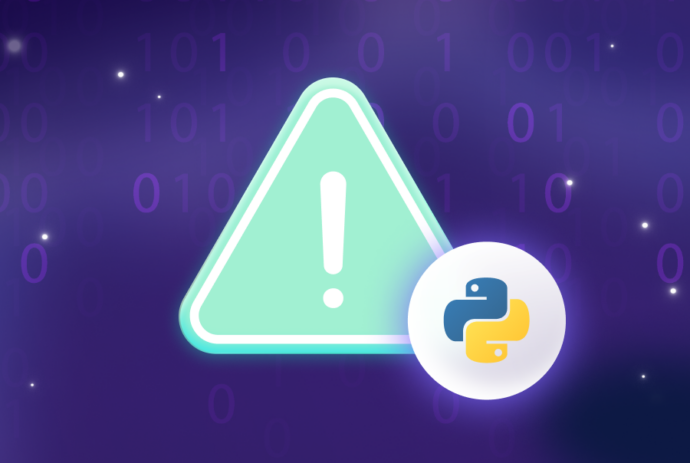
Exception handling in Python is often treated as an afterthought, tacked on at the end of development when things start breaking in production. Yet proper error handling is what separates robust applications from fragile ones that crumble at the first sign of unexpected input.
While it’s tempting to wrap everything in a generic try-except block and call it a day, thoughtful error handling can mean the difference between graceful degradation and catastrophic failure. Let’s dive into six practical techniques that will elevate your exception handling from basic error-catching to professional-grade defensive programming.
1. Keep your try blocks laser-focused
When handling exceptions in Python, the scope of your try blocks directly impacts how effectively you can diagnose and fix issues. Large, encompassing try blocks often mask the true source of errors and make debugging significantly more challenging.
Consider this problematic approach:
try: user = get_user_from_db() profile = process_user_data(user) send_notification(profile) except Exception as e: logger.error(f"Operation failed: {e}")
This code has several issues:
- It’s impossible to determine which operation actually failed
- All errors receive the same generic handling
- The error message lacks specific context
- Recovery options are limited since different errors are treated identically
Here’s how we can improve it:
try: user = get_user_from_db() except DatabaseError as e: logger.error(f"Database connection failed: {e}") return try: profile = process_user_data(user) except ValidationError as e: logger.error(f"User data invalid: {e}") return try: send_notification(profile) except NotificationError as e: logger.error(f"Notification delivery failed: {e}") return
Now, as you can notice:
- Each operation has its own error boundary
- Error messages contain operation-specific context
- Different types of failures can be handled appropriately
- Debugging becomes straightforward as the error source is immediately apparent
- Each operation can implement specific recovery strategies
The extra code is a worthwhile trade-off for the clarity and control it provides. While it might seem verbose, this pattern becomes invaluable as applications grow in complexity and when we’re debugging production issues.
2. Catch specific exceptions
Python’s exception hierarchy is extensive and well-designed, yet many developers fall into the trap of using overly broad exception handling. In the hierarchy, built-in exceptions are organized logically — from broad base classes to specific error types. Each exception type serves a distinct purpose that you can use to identify and handle specific failure scenarios appropriately.
For instance, when working with dictionaries, catch `KeyError` rather than `Exception`. When parsing strings to integers, catch `ValueError`instead of using a broad try-except block:
def process_user_data(data): try: user_id = data['id'] return db.get_user(user_id) except KeyError: logger.error("Missing required field: id") raise ValueError("User data must contain an id field") except ConnectionError: logger.error("Database connection failed") return None
The hierarchy also lets you catch multiple related exceptions elegantly:
try: value = int(user_input) except (TypeError, ValueError) as e: logger.error(f"Invalid input: {e}")
The key points here are:
- You should never use bare except clauses.
- Always avoid catching `Exception` unless you’re re-raising it.
3. Use context managers wisely
Context managers in Python shine when it comes to resource management, but many developers miss out on their potential. While they’re commonly associated with file operations, their scope extends far beyond basic file handling.
The real power of context managers lies in their ability to handle setup and cleanup operations automatically, whether your code executes successfully or raises an exception. This becomes particularly crucial when dealing with:
- Database connections and transactions
- Network sockets and API sessions
- Memory-intensive operations
- Thread locks and semaphores
- Temporary file management\
Here’s an example:
from contextlib import contextmanager import psycopg2 @contextmanager def db_transaction(): conn = psycopg2.connect(DATABASE_URL) try: yield conn conn.commit() except Exception: conn.rollback() raise finally: conn.close()
This pattern ensures proper resource cleanup even when errors occur. You can then use it elegantly in your code:
def update_user_profile(user_id, data): with db_transaction() as conn: cursor = conn.cursor() cursor.execute("UPDATE users SET profile = %s WHERE id = %s", (data, user_id))
Context managers particularly excel in production environments where resource leaks can cause significant issues. They enforce clean resource management patterns and make code more maintainable by centralizing setup and cleanup logic.
The idea is to identify operations that require paired setup and cleanup actions. Not every operation needs a context manager — reserve them for scenarios involving resource management or operations that need guaranteed cleanup.
4. Use Exception Groups for concurrent code
Python 3.11 introduced Exception Groups, a powerful feature that shines when handling multiple exceptions occurring simultaneously, especially in concurrent operations. Traditional exception handling falls short when dealing with parallel tasks that might fail independently.
def process_concurrent_tasks(): try: # Multiple operations that may fail simultaneously results = run_parallel_tasks() except* ExceptionGroup as eg: # Handle exceptions by type for exc in eg.exceptions: if isinstance(exc, ValueError): handle_value_error(exc) elif isinstance(exc, ConnectionError): handle_connection_error(exc)
Exception Groups provide several advantages. Now you can:
- Group related or unrelated exceptions logically
- Handle multiple errors without losing contextMaintain clean error hierarchies in concurrent codeEnable precise error handling based on exception types.
5. Add contextual notes to exceptions
The add_note() method, introduced in Python 3.11, lets you attach contextual breadcrumbs to exceptions as they bubble up through your application stack. This creates a richer debugging narrative without muddying the original error.
def process_user_data(user_id, data): try: validate_data(data) update_database(user_id, data) except ValueError as e: e.add_note(f"Error occurred while processing user {user_id}") e.add_note(f"Timestamp: {datetime.now()}") e.add_note(f"Data state: {data.get('status')}") raise
This approach offers several benefits:
- Preserves error integrity: The original exception remains intact, maintaining the precise error type and traceback.
- Contextual enrichment: Allows multiple contextual notes for comprehensive error reporting.
- Performance-conscious: Adding notes incurs minimal overhead, as they’re only formatted when the exception is printed.
6. Implement proper logging
When exceptions hit the fan in production, proper logging becomes your best friend. While many developers can be found slapping a print statement or a basic `logger.error()`, a thoughtful logging strategy can save countless debugging hours.
Here’s what they all miss — logging isn’t just about catching errors. Strategic logging helps track user behavior patterns, performance bottlenecks, and system health. When an exception occurs, your logs should tell a story about what led to the failure.
Try this setup, for instance:
import logging logging.basicConfig( level=logging.INFO, format='%(asctime)s - %(name)s - %(levelname)s - %(message)s' )
The secret sauce to exceptional logging lies in these key ingredients:
- Log levels that actually mean something (`INFO` isn’t just `DEBUG`’s cousin)
- Contextual information that helps reconstruct the error state
- Stack traces for exceptions (but only when they matter)
- Structured logging in production for easier parsing
- Log rotation to manage storage efficiently
In Conclusion
The key is finding the right balance between comprehensive error handling and code readability. Qodo can significantly enhance this process — its AI analyzes your code patterns and identifies potential edge cases where exceptions might occur. By integrating directly with your IDE, Qodo can suggest appropriate exception-handling patterns and even generate test cases that validate your error-handling logic. This means you can catch potential issues before they reach production, ensuring your exception handling is both comprehensive and properly tested.
All in all, keep your error handling focused, your logging informative, and your exceptions meaningful. With modern tools and best practices working together, you can build more resilient applications that gracefully handle whatever comes their way.