Implementing the Testing Pyramid in Your Development Workflows
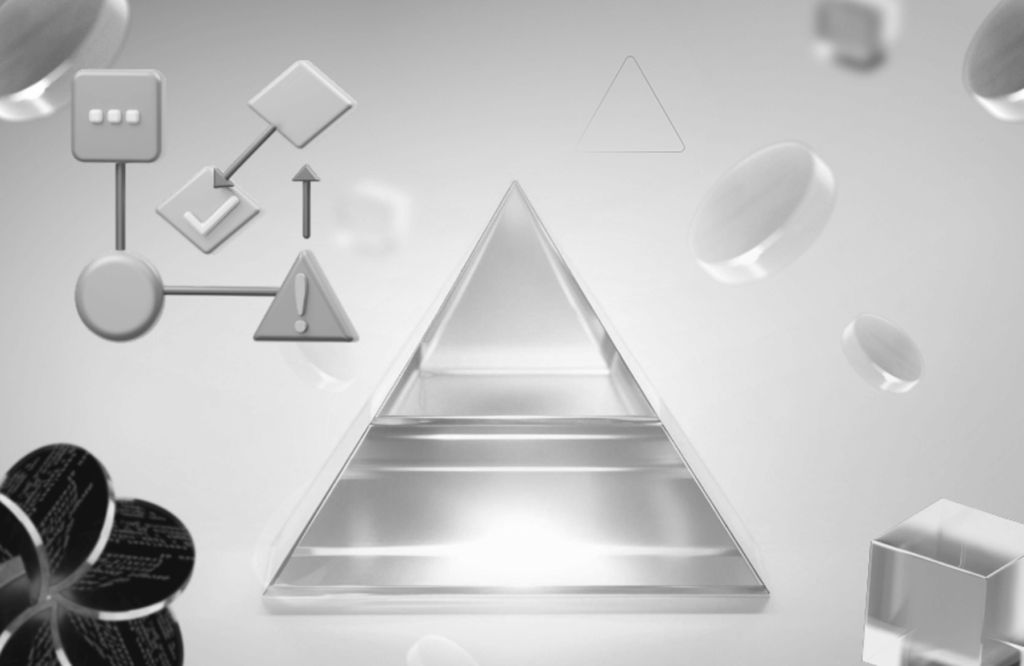
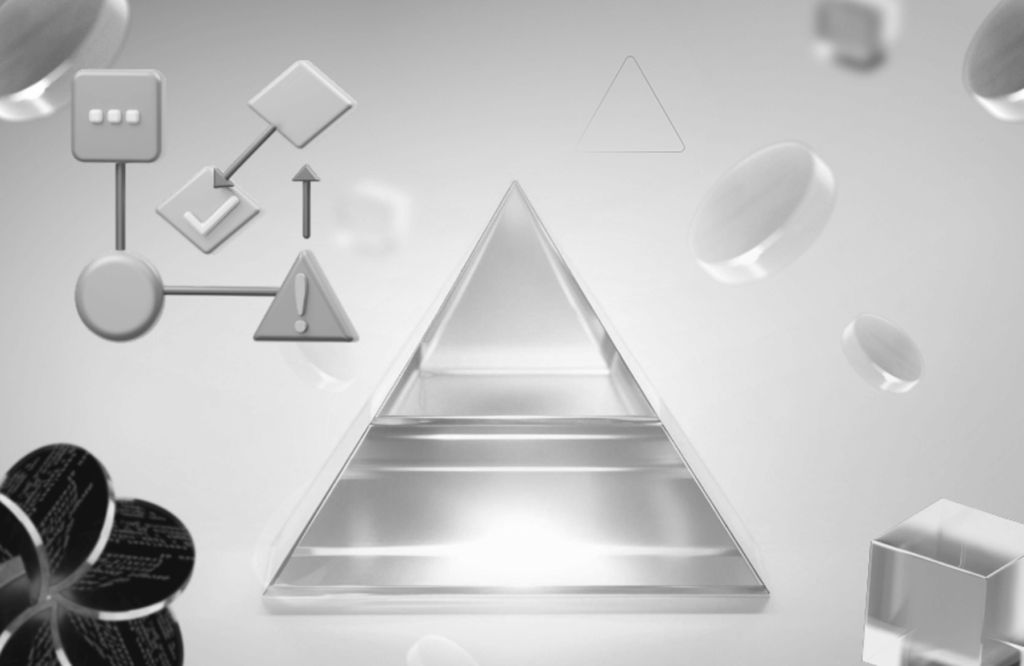
The Testing Pyramid serves as a guide for creating a balanced and efficient testing strategy, particularly in Agile environments. The Agile testing pyramid encourages teams to adopt a bottom-heavy approach, emphasizing thorough testing across three levels:
- Unit tests form the foundation. These tests validate the smallest parts of the application (like functions or methods) in isolation. They are fast to execute and easy to write.
- Service or integration tests focus on business logic. This level evaluates the interactions between different components or services. These tests are slower than unit tests but faster than end-to-end tests. They are also more complex, as they deal with multiple components.
- End-to-end (E2E) tests sit at the top and validate the complete flow of the application from the user’s perspective. They are the slowest and most costly to maintain. E2E tests check real user scenarios.
This model was first introduced by Mike Cohn in 2009 and helps teams prioritize faster, more reliable tests (like unit tests) while minimizing the slower, more brittle tests (like UI tests), ensuring that automation drives continuous feedback and early detection of defects. While manual testing remains valuable for exploratory purposes, the pyramid is designed to make automated testing the backbone of your quality strategy.
Why implement the Testing Pyramid?
The testing pyramid emphasizes the balance between unit tests, integration tests, and end-to-end tests. This structure helps teams focus their testing efforts on the most impactful areas, thus optimizing both time and resources. It provides several benefits:
- Cost efficiency
By focusing on a larger number of unit tests, you can catch bugs early when they are cheaper to fix. - Speed
Automated tests run faster when they are primarily unit tests, allowing for quicker feedback loops. - Clarity
The pyramid structure clarifies the relationships and purposes of different types of tests, making it easier for teams to understand where to invest their effort.
What is the modern Testing Pyramid?
Though Cohn’s original pyramid can seem overly simplistic today, given the advancements in development technologies, architectures, and tools, its core principles are still relevant. Modern frameworks, such as those for single-page applications (SPAs), highlight that UI tests don’t necessarily belong only at the top level-they can span across multiple levels.
Still, the testing pyramid is an excellent starting point for developing a custom testing model suited to your project. The pyramid’s layers can be adapted to fit any context or architecture by assigning specific roles and responsibilities to each level. To create your own testing pyramid model, follow these steps:
- Identify problem areas in your current testing strategy.
- Define the necessary types of tests based on your project needs.
- Establish target test coverage for each type.
- Evaluate the cost of each test type, considering your technology stack, business domain, architecture, and legacy code.
- Assign roles responsible for maintaining each level of the software testing pyramid.
Your testing pyramid might end up looking like this:
When building your testing pyramid, keep the following principles in mind:
- Test at the appropriate level
A unit test should validate code at the module level, not the API level. - Avoid redundancy
Higher-level tests, like E2E tests, should not replicate the logic already covered by lower-level tests. - Understand complexity
As you move up the pyramid, tests become more complex, expensive to develop, and fewer in number. - Prioritize feedback
Tests higher up the pyramid, while fewer, are more critical to business functionality, so longer feedback loops are acceptable but must still be efficient.
[blog-subscribe]
Steps to implement the Testing Pyramid
1. Assess your current testing strategy
Begin by analyzing your existing testing practices. Consider the following plan:
- Identify which levels of your testing pyramid are causing issues. Are there gaps in unit tests, too few integration tests, or unreliable UI tests? Investigate the root causes-whether it’s a lack of automation, insufficient test coverage, or inefficient test execution.
- Brainstorm solutions for each issue, prioritizing those that offer the greatest benefit in terms of risk reduction and problem elimination. Consider the impact of each solution on your team’s workflow and the quality of your software. Assess the resources-such as time, tools, or training-needed to implement these solutions effectively.
- Before you focus on improving any level of the pyramid, assess your available resources. This includes evaluating your team’s size, skill sets, workload, and level of engagement. Ensure that you have the necessary personnel, expertise, and capacity to implement the proposed changes without overwhelming the team.
2. Prioritize unit tests
Focus initially on building a strong base of unit tests (sometimes referred to as module testing).
Unit testing focuses on verifying the smallest atomic parts of an application’s code, such as classes, functions, or methods.
Example
Imagine your team is developing a calculator app that performs addition and subtraction. Each operation is implemented as a separate function. Testing each of these functions in isolation, without any dependencies, is an example of unit testing.
Benefits of unit testing
- Unit tests help catch bugs at the most fundamental level of code, targeting specific functions or methods.
- Since unit tests focus on individual components and are typically easier to write, update, and maintain.
- One of the biggest advantages is the speed at which unit tests run. Their quick execution allows for rapid feedback, making it easier to ensure that changes in the codebase don’t introduce new issues (regression testing).
Characteristics and best practices
- Always automated
Unit tests are consistently automated, allowing for rapid execution and continuous integration. The automated testing pyramid encourages a strategy where the majority of testing is done at the unit level in order to reduce the manual burden and speed up the overall testing process. - More frequent than other tests
There are always more unit tests compared to higher-level tests (like integration or E2E tests) because they target smaller, more granular parts of the system. - Independence
Unit tests are designed to run independently from other modules or the user interface. Their isolation ensures that external factors do not affect the outcomes. - Developer responsibility
In 99% of cases, developers write and run unit tests. When an error is found, no formal bug report is typically created. Instead, the developer fixes the bug immediately, reruns the tests, and continues this cycle until all tests pass.
At this level, developers (or automated testers) use the white-box testing approach. They have full visibility into the internal workings of the code, knowing exactly what inputs a function receives, what outputs it produces, and how it operates internally. This knowledge helps in crafting more precise and efficient tests.
3. Add integration tests
Once a solid unit test foundation is in place, start adding integration tests.
Integration tests verify the interactions between components that have already passed unit testing. These tests focus on how individual components work together, as well as how they integrate with external systems such as the operating system, services, databases, or hardware. Integration tests may also include API tests.
Characteristics
- No UI required
Integration testing typically does not require a user interface, as the interactions between components happen through interfaces like APIs or services. For example, you can test how a module communicates with a database or logs events on a server without needing a visual interface. - Functional and non-functional testing
Integration testing includes both functional checks (verifying that the system works as specified) and non-functional checks (e.g., performance and load testing across integrated components). - Gray or Black Box approach
Depending on the project, either a gray box (partial knowledge of internal workings) or black box (no knowledge of internals) approach may be used in integration testing.
Integration testing methods
For integration testing, you may follow one of the three testing methods described below.
- Bottom-up integration starts by testing the smallest, lower-level modules first and gradually integrating them into larger modules for testing. For example, in a social media app where a user can publish a photo, the **photo uploader** module consists of smaller parts like compression and server upload. Once the uploader components are tested, they are combined with the **photo publisher** module, which consists of verification and access control. The process continues, testing each larger integration in sequence.
- Top-down integration begins with higher-level modules, testing them first and simulating the behavior of lower-level modules that haven’t been developed yet by using stubs. For instance, when testing the interaction between a loader and a publisher, a simulated image (a “stub”) may be passed from the loader to the publisher, mimicking the behavior of the lower-level compression function. Lower-level modules are integrated and tested later.
- In Big Bang integration, all developed modules are integrated into the system simultaneously and tested as a whole. While this method can save time by testing everything at once, it often makes it more difficult to pinpoint the source of any issues, as failures could come from any of the interconnected components.
Developers and testers work closely during integration testing. Developers typically write integration tests and monitor the results, while testers may assist in designing tests or analyzing failures during regression testing. This collaboration ensures that any issues found during integration are addressed promptly, keeping the development process agile and efficient.
4. Implement End-to-End testing
E2E testing focuses on validating that the software meets all business requirements and functions as intended in real-world scenarios. Unlike tests at the system level that check specific functionality, E2E or acceptance tests verify the software as a whole, ensuring it works as expected from the perspective of both the end user and the client.
Characteristics
- Requirement validation
Acceptance tests validate that the software meets the specified requirements and performs as intended across various use cases. These tests are designed based on user stories, business requirements, and real-world workflows, ensuring that the software is fit for purpose. - Acceptance criteria
These tests are conducted only after the product has reached a required level of quality. Additionally, the acceptance plan-which outlines the set of scenarios, tests, execution date, and success criteria-must be reviewed and approved by the customer or stakeholders before testing begins.
Acceptance testing can be done either internally by the development team or by external parties, such as the client or end users. It’s important to note that these tests are not always executed by professional testers; often, stakeholders or even the customer themselves will run the acceptance tests to validate the software against their expectations.
Challenges of E2E tests
- Complexity
E2E tests simulate full user journeys, which makes them complex to write, maintain, and automate. - Time-consuming
These tests often take longer to execute compared to lower-level tests because they test the entire system. When running E2E tests as part of regression testing, they can slow down the overall test cycle due to their complexity and runtime. - Higher costs
Developing and maintaining E2E tests requires more effort and resources, making them more expensive compared to other test types.
Given these challenges, it is important to limit the number of E2E tests. Focus on the most critical user journeys and business requirements to minimize maintenance costs while ensuring key functionalities are covered.
Implementing the testing pyramid in your development workflows can significantly improve your testing strategy, leading to faster releases and higher-quality software. By understanding the structure of the pyramid and following a structured approach to integrate it into your workflows, your team can streamline workflows and deliver exceptional software products that meet users’ needs and expectations.